Welcome to Laravel Pagination Search Filter Results Example Tutorial.
In this tutorial, you will learn to set the data in the pagination format in laravel.
You will also learn how to add search filter functionality in the pagination to search specific data.
We will represent the data in the tabular manner with limited number of rows.
For integrating the laravel pagination search filter, we will use laravel scout package.
Final Results Of Laravel Pagination Search Example
Below images represents the final screens of this tutorial.
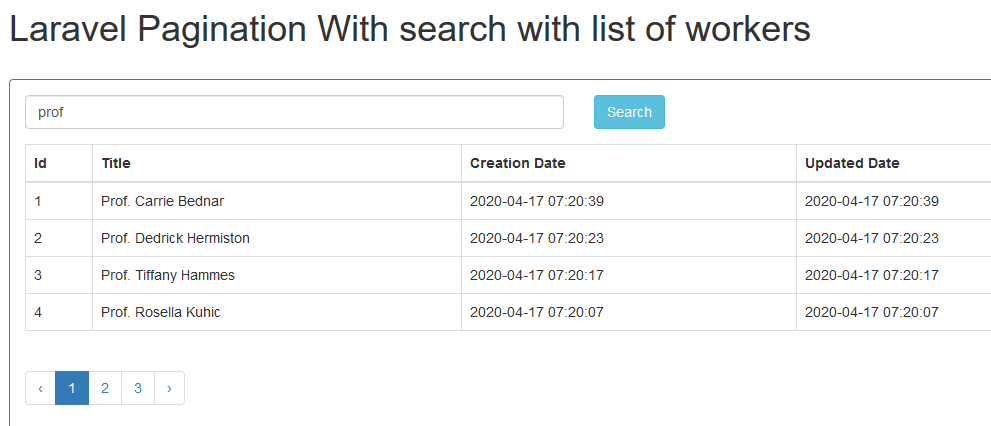
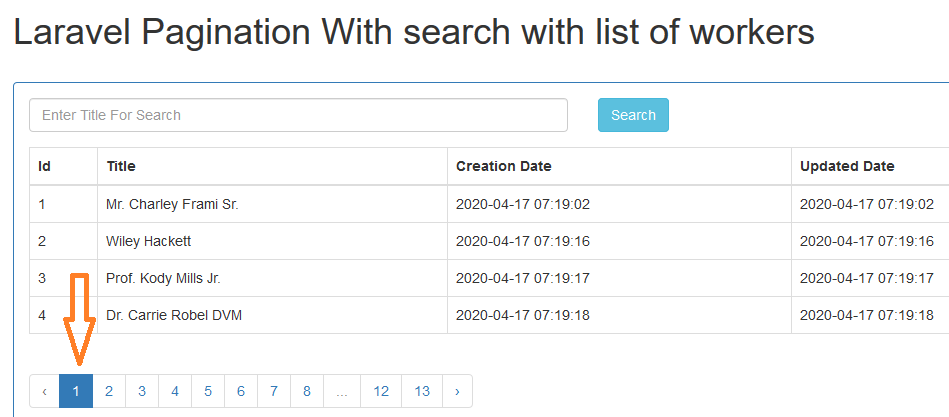
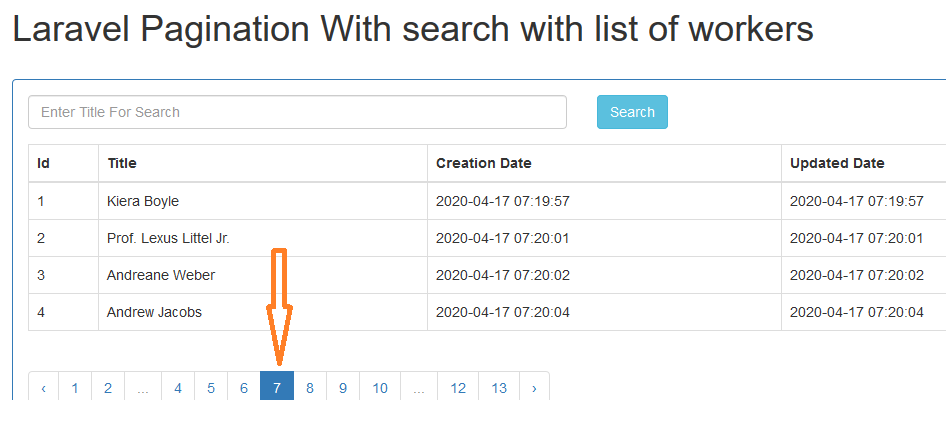
Now follow all the below steps to produce the example like the above images.
Step 1. Project with Database
Use the following command to generate the new laravel project.
laravel new larapaginationsearch
After this, open your database management tool like phpMyAdmin or sequel pro.
Make a new database and give it a name like “larapaginationsearch“.
Now let us connect our database with the laravel project. For that, open your project in the code editor.
Now go to your .env file. Inside this .env file, there must be some database configuration lines like the below
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=larapaginationsearch DB_USERNAME=root DB_PASSWORD=
Enter details like database name, username and password.
Once you entered this details, you have successfully connected your database with the project.
Step 2. Some Packages
We need to have two packages in this example to implement search functionality in pagination.
First of all, fire the below command
composer require laravel/scout
Above line will help us to integrate the scout package in our laravel project.
Now let us modify the config->app.php file. In the app.php file, find the array “providers“.
You should add the following line in this config->app.php file.
Laravel\Scout\ScoutServiceProvider::class,
So your providers array should look like the below code snippet
'providers' => [ /* * Laravel Framework Service Providers... */ Illuminate\Auth\AuthServiceProvider::class, Illuminate\Broadcasting\BroadcastServiceProvider::class, Illuminate\Bus\BusServiceProvider::class, Illuminate\Cache\CacheServiceProvider::class, . . . Laravel\Scout\ScoutServiceProvider::class,
After this, we need to publish the modification of the app.php file.
For this, run the below command in your command prompt
php artisan vendor:publish --provider="Laravel\Scout\ScoutServiceProvider"
So our work related to scout package is now complete.
Now it is time to add algolia package in the project.
For this, run the below command
composer require algolia/algoliasearch-client-php
After the successful implementation of the above command, we need to generate APP ID and ADMIN API KEY from the algolia.
For this go to the : https://www.algolia.com/apps
If you do not have account with algolia then create one.
Now go to the screen like the below image.
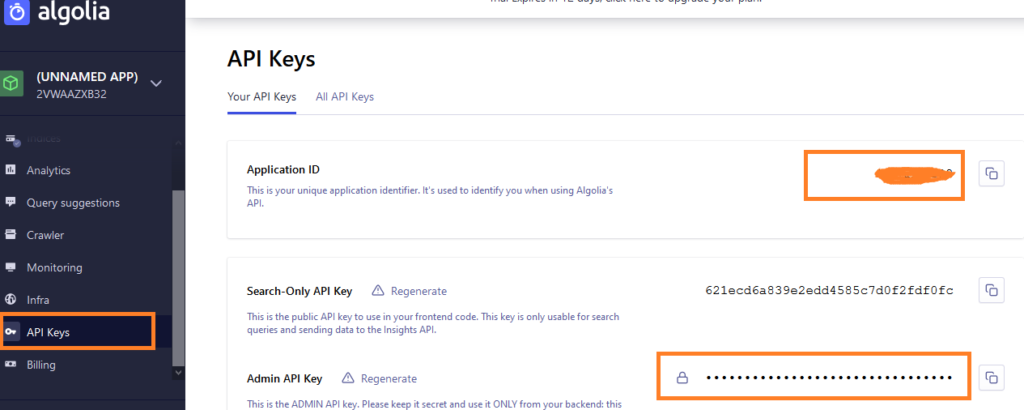
You can find the Application ID and Admin API Key as per the shown in the above image.
We have to put these Application ID and Admin API Key in the ,env file of our laravel project.
So open your .env file and write the below lines in it
ALGOLIA_APP_ID=2V...... ALGOLIA_SECRET=886e1e.....
Step 3. Generating Data
Now it is time to generate some data so that we can use them to set in pagination method.
For this let us first create one table in the database. So run the below command
php artisan make:migration create_workers_table
Go to the app/database/migration/timestamp_create_workers_table.php directory and write down the below coding in it
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateWorkersTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('workers', function (Blueprint $table) { $table->increments('id'); $table->string('title'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('workers'); } }
Above file have one function called up() which holds the column names.
There are three columns : id ,title and timestamp.
Now we also need to create the model class for the worker.
So go ahead and fire the below command
php artisan make:model Worker
Above command will make a new php file called Worker.php inside the app folder.
Now open this Worker.php file and add the following coding lines in it
<?php namespace App; use Illuminate\Database\Eloquent\Model; use Laravel\Scout\Searchable; class Worker extends Model { // use Searchable; public $fillable = ['title']; public function searchableAs() { return 'workers_order'; } }
in the above file, make sure that you have added ” use Laravel\Scout\Searchable; ” line as well as “ use Searchable; ” lines.
These lines are important to implement the search filter on the Worker model.
Till now we do not have created the workers table into the database.
So go through the below command to make this table,
php artisan migrate
Now check in your database management tool, you must find the table called “worker“
Step 4. Final Files
First of all, let us write the controller file. So use the below command
php artisan make:controller WorkerController
Now open the file : app/Http/Controllers/WorkerController.php
Put the below coding snippet inside the file WorkerController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Http\Requests; use App\Worker; class WorkerController extends Controller { // public function index(Request $request) { if($request->has('titlesearch')){ $workers = Worker::search($request->titlesearch) ->paginate(4); }else{ $workers = Worker::paginate(4); } return view('search',compact('workers')); } }
Look at the index() function in the above controller file.
In this function, compiler will first check one if condition.
If request has the id as “titlesearch” then if condition is true.
Here, compiler will search the results using the search query. After this, it will paginate the data with four rows in one page.
When if condition is false, compiler will simply fetch all the data and it will display them with four rows in single page.
Now it is time to setup the routes of this example.
Open the app->routes->web.php file. and add the below line in it
Route::get('workers-orders', ['as'=>'workers-orders','uses'=>'WorkerController@index']);
After the route, we need to write the blade view file.
For this, you need to navigate to the app->resources->views directory and make a new file with the name like “search.blade.php“
search.blade.php file should contain the following source code snippet
<!DOCTYPE html> <html> <head> <title>Laravel Pagination With search with list of workers</title> <link rel="stylesheet" type="text/css" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> </head> <body> <div class="container"> <h1>Laravel Pagination With search with list of workers</h2><br/> <div class="panel panel-primary"> <div class="panel-body"> <form method="GET" action="{{ route('workers-orders') }}"> <div class="row"> <div class="col-md-6"> <div class="form-group"> <input type="text" name="titlesearch" class="form-control" placeholder="Enter Title For Search" value="{{ old('titlesearch') }}"> </div> </div> <div class="col-md-6"> <div class="form-group"> <button class="btn btn-info ">Search</button> </div> </div> </div> </form> <table class="table table-bordered"> <thead> <th>Id</th> <th>Title</th> <th>Creation Date</th> <th>Updated Date</th> </thead> <tbody> @if($workers->count()) @foreach($workers as $key => $worker) <tr> <td>{{ ++$key }}</td> <td>{{ $worker->title }}</td> <td>{{ $worker->created_at }}</td> <td>{{ $worker->updated_at }}</td> </tr> @endforeach @else <tr> <td colspan="4">There are no data.</td> </tr> @endif </tbody> </table> {{ $workers->links() }} </div> </div> </div> </body> </html>
Above blade file represent the view structure of this example.
We have taken one form and we will display the data into the tabular manner using the above blade file.
Now we need to add dummy data into our database table to test the results.
So open the app->database->factories->UserFactory.php file and add the below lines in it
$factory->define(Worker::class, function (Faker $faker) { return [ 'title' => $faker->name, ]; });
You also need to add ” use App\Item; ” at the beginning of the UserFactory.php file.
Final code for UserFactory.php file is like the following
<?php /** @var \Illuminate\Database\Eloquent\Factory $factory */ use App\User; use App\Worker; use Faker\Generator as Faker; use Illuminate\Support\Str; /* |-------------------------------------------------------------------------- | Model Factories |-------------------------------------------------------------------------- | | This directory should contain each of the model factory definitions for | your application. Factories provide a convenient way to generate new | model instances for testing / seeding your application's database. | */ $factory->define(User::class, function (Faker $faker) { return [ 'name' => $faker->name, 'email' => $faker->unique()->safeEmail, 'email_verified_at' => now(), 'password' => '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', // password 'remember_token' => Str::random(10), ]; }); $factory->define(Worker::class, function (Faker $faker) { return [ 'title' => $faker->name, ]; });
Now run the below command
php artisan serve
right after the above command, run the below command
factory(App\Worker::class, 50)->create();
Above command should add the 50 dummy data rows to your database.
Now run the command “php artisan serve” to run the project.
Finally, our laravel pagination search tutorial is over.