AJAX = Asynchronous JavaScript and XML.
Ajax is ideal for update the part in webpage without reload the whole page.
It is used to communicate between webpage and web-server.
Here we create a search box using ajax with PHP script.
User enter the course name into search box and get the suggestion course name which alphabet he/she enters.
User get the hint how many courses are there starting with the given letter.
First create search page with AJAX and PHP script.
Here we create one search.php page.
<html>
<head>
<script>
function displayHint(str) {
if (str.length == 0) {
document.getElementById("Hint").innerHTML = "";
return;
} else {
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("Hint").innerHTML = this.responseText;
}
};
xmlhttp.open("GET", "hint.php?getparameter=" + str, true);
xmlhttp.send();
}
}
</script>
</head>
<body>
<p><b>Enter the Course name:</b></p>
<form action="">
<label for="cname">Course name:</label>
<input type="text" id="cname" name="cname" onkeyup="displayHint(this.value)">
</form>
<p>Show Suggestions: <span id="Hint"></span></p>
</body>
</html>
For the above code we got the output something like this –
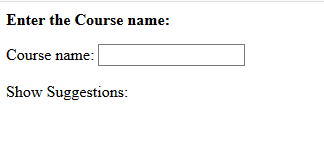
Function displayHint() is executed when the user types a character in the input field.
This function is triggered by onkeyup event.
Now, create a file named hint.php
This file contains an array of course names.
When user enters an letter in search box the course name will be triggered according to that latter from this hint.php page.
hint.php
<?php
// Array with names
$a[] = "C";
$a[] = "C++";
$a[] = "PHP";
$a[] = "JAVA";
$a[] = "Android";
$a[] = "IOS";
$a[] = "Codeignitor";
$a[] = "Laravel";
$a[] = "Magento";
$a[] = ".NET";
$a[] = "Perl";
$a[] = "Ruby";
$a[] = "Python";
$a[] = "Bootsrap";
$a[] = "SQL";
$a[] = "HTML";
$a[] = "CSS";
$a[] = "Angular JS";
$a[] = "Jquery";
$a[] = "React";
$a[] = "Ajax";
$a[] = "Node.JS";
$a[] = "Json";
$a[] = "Dart";
$a[] = "Scala";
$a[] = "Kotlin";
$a[] = "Rust";
$a[] = "C#";
$a[] = "Delphi";
$a[] = "Lua";
// get the parameter from URL
$getparameter = $_REQUEST["getparameter"];
$hint = "";
// lookup all hints from array if $getparameter is different from ""
if ($getparameter !== "") {
$getparameter = strtolower($getparameter);
$len=strlen($getparameter);
foreach($a as $name) {
if (stristr($getparameter, substr($name, 0, $len))) {
if ($hint === "") {
$hint = $name;
} else {
$hint .= ", $name";
}
}
}
}
// Output "no suggestion" if no hint was found or output correct values
echo $hint === "" ? "no suggestion" : $hint;
?>
Here is the output which is showing the search result.
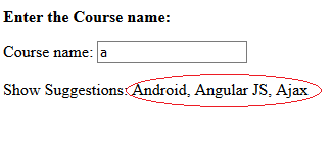