Hello coders, you will learn to integrate elasticsearch in laravel today.
When you are displaying the data into the tabular form to the user, you need to provide search filter feature for user for his comfort.
For search feature, we can use elasticsearch to make the process easy.
Elasticsearch is the library or search engine which provides full support for text based search.
Using elasticsearch in the laravel, we can achieve high scalable search functionality in our laravel app.
So let us integrate the elasticsearch in php laravel.
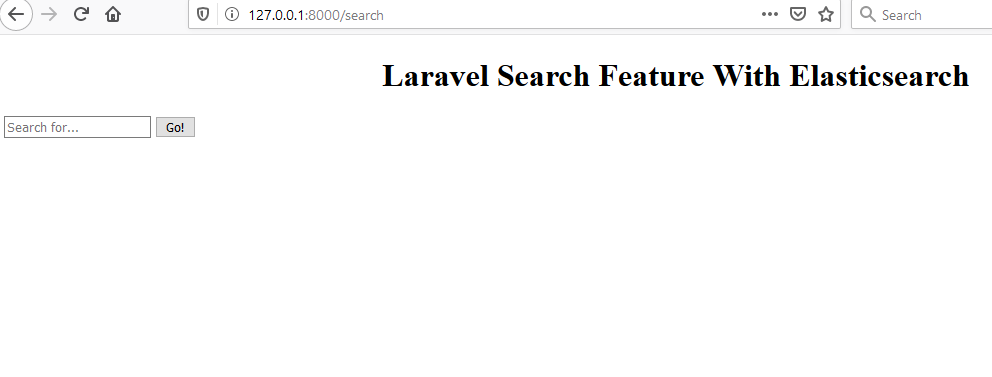
Step 1. Starting Elasticsearch
To use the elasticsearch, we need to first install it on our machine.
Here is the tutorial on how to install elasticsearch on mac.
Also you can install elasticsearch on windows.
For that, go to command line or terminal and hit the below command
brew tap elastic/tap
Now, run the following command
brew install elastic/tap/elasticsearch-full
Finally, start the elasticsearch on your computer
brew services start elasticsearch
After the above command, we have successfully installed the elasticsearch on our mac apple machine and also we have started the elasticsearch service.
Step 2. New Laravel Project With Database
To create a fresh laravel project, fire the below command
laravel new laraElastic
Now open your laravel project in your code editor. Inside code editor, open composer.json file.
Inside this composer.json file, find the following lines
"require": { "php": ">=7.1.3", "fideloper/proxy": "~4.0", "laravel/framework": "5.6.*", "laravel/tinker": "~1.0", "laravelcollective/html": "^5.6.39", "elasticquent/elasticquent": "dev-master" },
In the above snippet, last line is for elasticsearch. You need to add this last line in your composer.json file.
After this, you need to update the composer. You should run the following command to update the composer.
composer update
Step 3. Elasticsearch Configuration
Open config->app.php file. Inside it, add the line “Elasticquent\ElasticquentServiceProvider::class” in “providers” array
'providers' => [ /* * Laravel Framework Service Providers... */ Illuminate\Auth\AuthServiceProvider::class, Illuminate\Broadcasting\BroadcastServiceProvider::class, Illuminate\Bus\BusServiceProvider::class, . . . Elasticquent\ElasticquentServiceProvider::class,
Now in the array “aliases“, add the line ” ‘Es’ => Elasticquent\ElasticquentElasticsearchFacade::class,”
'aliases' => [ 'App' => Illuminate\Support\Facades\App::class, 'Artisan' => Illuminate\Support\Facades\Artisan::class, 'Auth' => Illuminate\Support\Facades\Auth::class, . . . 'Es' => Elasticquent\ElasticquentElasticsearchFacade::class,
After this, we have to publish the elasticsearch configuration. You need do that by firing the below command
php artisan vendor:publish --provider="Elasticquent\ElasticquentServiceProvider"
Now go to the config file at app >> config >> elastiquent.php
Inside this file, find the following line
'default_index' => 'workers',
I have updated the value of “default_index” to workers.
Step 4. Making Migration With Model
Open your command prompt and hit the below command
php artisan make:migration create_workers_table
Above files will help us to make migration file for Workers table.
After this command, navigate to the app->database->migrations folder. Inside migrations folder, you will find a new file which may have name like timestamp_create_workers_table.php file.
Add the below code snippet inside this imestamp_create_workers_table.php file,
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateWorkersTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('workers', function (Blueprint $table) { $table->bigIncrements('id'); $table->string('name'); $table->text('country'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('workers'); } }
up() function in the above code gives idea regarding the column names of the workers table.
Now run the below command in terminal or command prompt
php artisan migrate
This command will create the workers table into the database.
To create the Worker model class, run the following command
php artisan make:model Worker
Now you should navigate to the app->Worker.php file and write down the below source in it.
<?php namespace App; use Illuminate\Database\Eloquent\Model; use Elasticquent\ElasticquentTrait; class Worker extends Model { // public $table = "workers"; }
Now navigate to the app->database->factories->UserFactory.php file.
You need to add the following source lines in UserFactory.php file.
<?php /** @var \Illuminate\Database\Eloquent\Factory $factory */ use App\User; use App\Worker; use Faker\Generator as Faker; use Illuminate\Support\Str; /* |-------------------------------------------------------------------------- | Model Factories |-------------------------------------------------------------------------- | | This directory should contain each of the model factory definitions for | your application. Factories provide a convenient way to generate new | model instances for testing / seeding your application's database. | */ $factory->define(User::class, function (Faker $faker) { return [ 'name' => $faker->name, 'email' => $faker->unique()->safeEmail, 'email_verified_at' => now(), 'password' => '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', // password 'remember_token' => Str::random(10), ]; }); $factory->define(Worker::class, function (Faker $faker) { return [ 'name' => $faker->name, 'country' => $faker->country, ]; });
Look at the last few lines in the above code.
These lines are defining the factory code to generate the fake data into the workers table.
After this, again go to command tool and hit the below command
php artisan tinker
and after the above command, type the below lines in command tool only
factory(App\Worker::class, 50)->create();
Above line will add the dummy 50 rows into the workers table.
Step 5. Controller and Blade Files
Again, use the below command first
php artisan make:controller WorkersController
It will create the controller file. Go to app->Http->Controllers->WorkersController.php file and write the following lines in it
namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Http\Requests; use App\Worker; class WorkersController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index(Request $request) { if($request->has('search')){ $items = Worker::search($request->input('search'))->toArray(); } return view('WorkerSearch',compact('workers')); } }
Now let us add the route line. Go to the app/Http/routes.php file and add the following line in it
Route::get('WorkerSearch', 'WorkersController@index');
Time to make blade view files for our project.
First of all, go to app->resources->views directory. Inside views folder, make a new file and name it like “layout.blade.php” and add the blow code in it
</<!DOCTYPE html> <html> <head> <link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com /ajax/libs/bulma/0.7.2/css/bulma.css"> </head> <body> <div class="container"> @yield('content') </div> </html>
Now in the views folder, make a new file named WorkerSearch.blade.php
Inside this WorkerSearch.blade.php file, write the below lines
@extends('layouts.app') @section('content') <div class="row"> <div class="col-md-8 col-md-offset-2"> <h1 class="text-primary" style="text-align: center;">Laravel 5 Search Using Elasticsearch</h1> </div> </div> <div class="container"> <div class="panel panel-primary"> <div class="panel-heading"> <div class="row"> <div class="col-lg-6"> {!! Form::open(array('method'=>'get','class'=>'')) !!} <div class="input-group"> <input name="search" value="{{ old('search') }}" type="text" class="form-control" placeholder="Search for..."> <span class="input-group-btn"> <button class="btn btn-default" type="submit">Go!</button> </span> </div><!-- /input-group --> {!! Form::close() !!} </div><!-- /.col-lg-6 --> </div><!-- /.row --> </div> <div class="panel-body"> <div class="row"> <div class="col-lg-6"> @if(!empty($items)) @foreach($items as $key => $value) <h3 class="text-danger">{{ $value['title'] }}</h3> <p>{{ $value['description'] }}</p> @endforeach @endif </div> </div> </div> </div> </div> </div>
Now run our project using the command “php artisan serve“
That is all about this example.