Topic of the today is Laravel Scout Search Filter Algolia Tutorial Example.
You will learn how to use scout in laravel to implement the text based search filter functionality.
We will see regarding the algolia scout search integration in the laravel framework.
Algolia provides search related functions which we can directly use in our laravel project.
We will fetch the row data from the MySQL database and then we will preview then in the tabular form.
User will be able to enter the search query and we will give the results based on the user’s search query.
We will filter the data by using the scout and algolia search features.
Laravel Scout Search Output
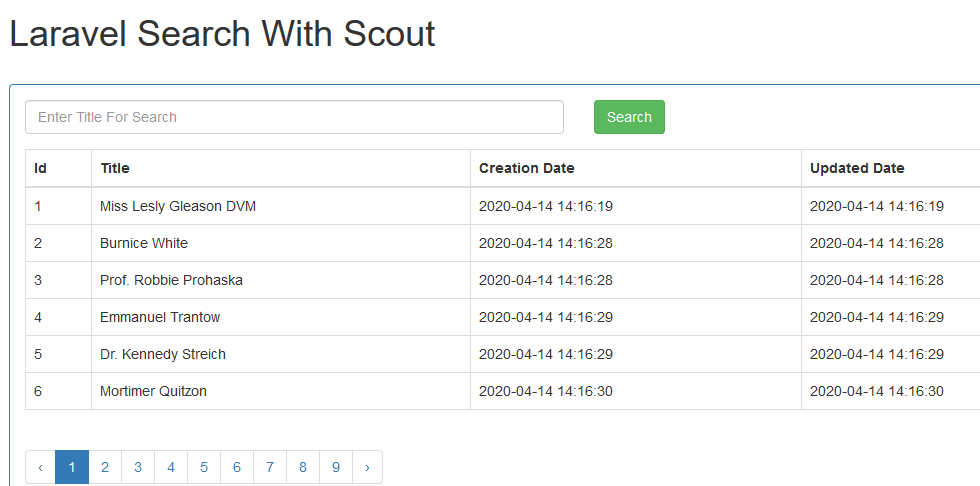
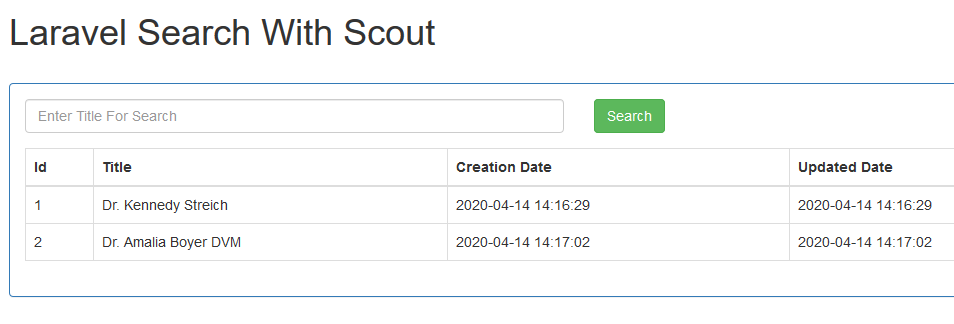
Now follow all the below steps to complete the example.
Step 1. New Project with Database
Open up your terminal or command prompt and hit the following command
laravel new larascout
Above command will create new laravel project.
Now let us make a new MySQL database. Open your database management tool like phpMyAdmin or sequel pro.
Manually add new database and set the name of the database as “larascout“
Now let us connect our laravel project and MySQL database with each other.
For this, open laravel project in code editor tool like sublime text, visual studio code or which ever you like.
Now open your .env file and find the below configuration lines for MySQL database.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=larascout DB_USERNAME=root DB_PASSWORD=
You need to enter above details as per your machine’s configuration.
I do not have set any password and my username is root. Also add the name of the database.
Step 2. Integrating Packages
Now it’s time to add required packages in our laravel project.
In your command prompt, fire the following command
composer require laravel/scout
Above command will simply integrate the scout package into our laravel project.
Now it is time to update the configuration for scout.
Navigate to the config/app.php file and find the array “provider“
In this “provider” array, add the line “Laravel\Scout\ScoutServiceProvider::class,”
See the below code snippet for more reference.
'providers' => [ /* * Laravel Framework Service Providers... */ Illuminate\Auth\AuthServiceProvider::class, Illuminate\Broadcasting\BroadcastServiceProvider::class, Illuminate\Bus\BusServiceProvider::class, Illuminate\Cache\CacheServiceProvider::class, . . . Laravel\Scout\ScoutServiceProvider::class,
Now we need to publish the configuration that we have just updated in the app.php file.
For publishing, run the following command
php artisan vendor:publish --provider="Laravel\Scout\ScoutServiceProvider"
After the scout, let us integrate algolia library. For this, run the below command
composer require algolia/algoliasearch-client-php
After the successful integration of algolia, we need to generate APP ID and Admin API KEY.
For this, go to the algolia dashboard. If you do not have any account with algolia then create one and go to the https://www.algolia.com/apps
From the left side bar, click on “API Keys” tab then you should see the following screen,
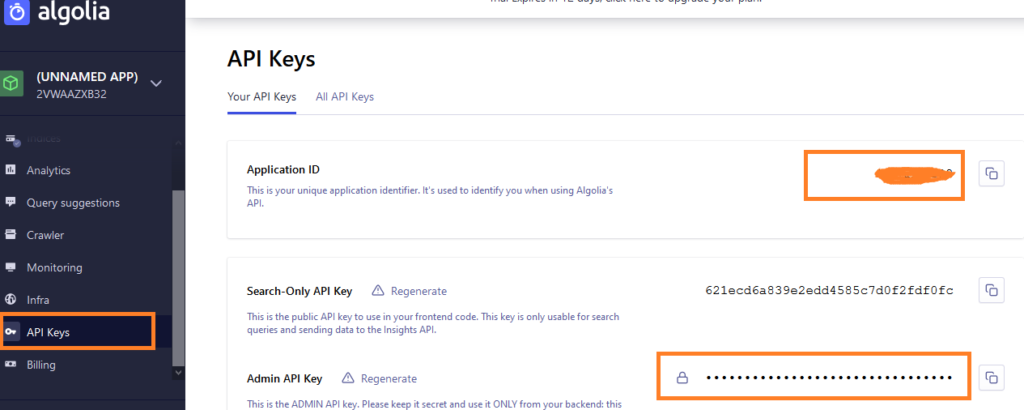
You can find APP ID and Admin API KEY for algolia from your screen like above image.
Now open your .env file and add the below lines in it
ALGOLIA_APP_ID=2VW...... ALGOLIA_SECRET=886e1ef........
Step 3. Creating Table in Database
You need to run the following in the command line
php artisan make:migration create_items_table
Above command will create one migration file.
Navigate to the app/database/migration/timestamp_create_items_table.php file and write down the following coding lines in it
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateItemsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('items', function (Blueprint $table) { $table->increments('id'); $table->string('title'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('items'); } }
Look at the up() function in the above code snippet.
This function includes the column names of the items table. There are three lines for it : id, title and timestamps.
Now let us create the model class for our items table. For this, run the below command
php artisan make:model Item
Now navigate to the app->Item.php file. Add the below coding lines in this file.
<?php namespace App; use Illuminate\Database\Eloquent\Model; use Laravel\Scout\Searchable; class Item extends Model { // use Searchable; public $fillable = ['title']; /** * Get the index name for the model. * * @return string */ public function searchableAs() { return 'items_index'; } }
In the above model file, make sure that you have added two below lines as per shown in the above code snippet
use Laravel\Scout\Searchable; use Searchable;
Once you have created migration and model files, let us create the items table.
For this, just run the below command.
php artisan migrate
Now check out your database. You should find the new table with the name as “items“
Step 4. Controller, Route and Blade
First of all, run the following command in command prompt
php artisan make:controller ItemSearchController
Above command will make one file at the location : app/Http/Controllers/ItemSearchController.php
Inside this ItemSearchController.php file, write the following coding lines
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Http\Requests; use App\Item; class ItemSearchController extends Controller { // public function index(Request $request) { if($request->has('titlesearch')){ $items = Item::search($request->titlesearch) ->paginate(6); }else{ $items = Item::paginate(6); } return view('search',compact('items')); } }
Look at the index() method in the above code block.
This method has one if condition.
When the if condition is correct, compiler will filter the data using the search query.
When if condition is false, compiler will simply show all the data.
Now let us set the routes for this example.
For this, navigate to the app->routes->web.php file.
Write down the following two route lines in this web.php file.
Route::get('items-lists', ['as'=>'items-lists','uses'=>'ItemSearchController@index']);
Now go to the app->resources->views directory. Inside views folder, make a new file and give it a name as search.blade.php
Add the below source snippet in the search.blade.php file.
<!DOCTYPE html> <html> <head> <title>Laravel scout search example</title> <link rel="stylesheet" type="text/css" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> </head> <body> <div class="container"> <h1>Laravel Search With Scout</h2><br/> <form method="POST" action="{{ route('create-item') }}" autocomplete="off"> @if(count($errors)) <div class="alert alert-danger"> <strong>Nop!</strong> There were some problems with your input. <br/> <ul> @foreach($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif <input type="hidden" name="_token" value="{{ csrf_token() }}"> </form> <div class="panel panel-primary"> <div class="panel-body"> <form method="GET" action="{{ route('items-lists') }}"> <div class="row"> <div class="col-md-6"> <div class="form-group"> <input type="text" name="titlesearch" class="form-control" placeholder="Enter Title For Search" value="{{ old('titlesearch') }}"> </div> </div> <div class="col-md-6"> <div class="form-group"> <button class="btn btn-success">Search</button> </div> </div> </div> </form> <table class="table table-bordered"> <thead> <th>Id</th> <th>Title</th> <th>Creation Date</th> <th>Updated Date</th> </thead> <tbody> @if($items->count()) @foreach($items as $key => $item) <tr> <td>{{ ++$key }}</td> <td>{{ $item->title }}</td> <td>{{ $item->created_at }}</td> <td>{{ $item->updated_at }}</td> </tr> @endforeach @else <tr> <td colspan="4">There are no data.</td> </tr> @endif </tbody> </table> {{ $items->links() }} </div> </div> </div> </body> </html>
Now our coding related work is complete. To run the example, we need some dummy data.
So navigate to the app->database->factories->UserFactory.php file.
Inside this UserFactory.php file, add the below code block
$factory->define(Item::class, function (Faker $faker) { return [ 'title' => $faker->name, ]; });
You also need to add ” use App\Item; ” at the beginning UserFactory.php file.
Below is the full code snippet for UserFactory.php file .
<?php /** @var \Illuminate\Database\Eloquent\Factory $factory */ use App\User; use App\Item; use Faker\Generator as Faker; use Illuminate\Support\Str; /* |-------------------------------------------------------------------------- | Model Factories |-------------------------------------------------------------------------- | | This directory should contain each of the model factory definitions for | your application. Factories provide a convenient way to generate new | model instances for testing / seeding your application's database. | */ $factory->define(User::class, function (Faker $faker) { return [ 'name' => $faker->name, 'email' => $faker->unique()->safeEmail, 'email_verified_at' => now(), 'password' => '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', // password 'remember_token' => Str::random(10), ]; }); $factory->define(Item::class, function (Faker $faker) { return [ 'title' => $faker->name, ]; });
After this, fire the following command
php artisan tinker
Then hit the below command
factory(App\Item::class, 50)->create();
When you run the above command, you should see the dummy data records in your command or terminal window.
Now go to your database and you should find 50 dummy rows of data inside the items table.
Now to run the example, run the below command
php artisan serve
Then in your browser, open the URL : http://127.0.0.1:8000/items-lists
So that is all about this laravel search functionality with scout and algolia example.